The __syncthreads()
command is a block level synchronization barrier. That means it is safe to be used when all threads in a block reach the barrier. It is also possible to use __syncthreads()
in conditional code but only when all threads evaluate identically such code otherwise the execution is likely to hang or produce unintended side effects [4].
Example of using __syncthreads()
: (source)
__global__ void globFunction(int *arr, int N) { __shared__ int local_array[THREADS_PER_BLOCK]; //local block memory cache int idx = blockIdx.x* blockDim.x+ threadIdx.x; //...calculate results local_array[threadIdx.x] = results; //synchronize the local threads writing to the local memory cache __syncthreads(); // read the results of another thread in the current thread int val = local_array[(threadIdx.x + 1) % THREADS_PER_BLOCK]; //write back the value to global memory arr[idx] = val; }
To synchronize all threads in a grid currently there is not native API call. One way of synchronizing threads on a grid level is using consecutive kernel calls as at that point all threads end and start again from the same point. It is also commonly called CPU synchronization or Implicit synchronization. Thus they are all synchronized.
Example of using this technique (source):
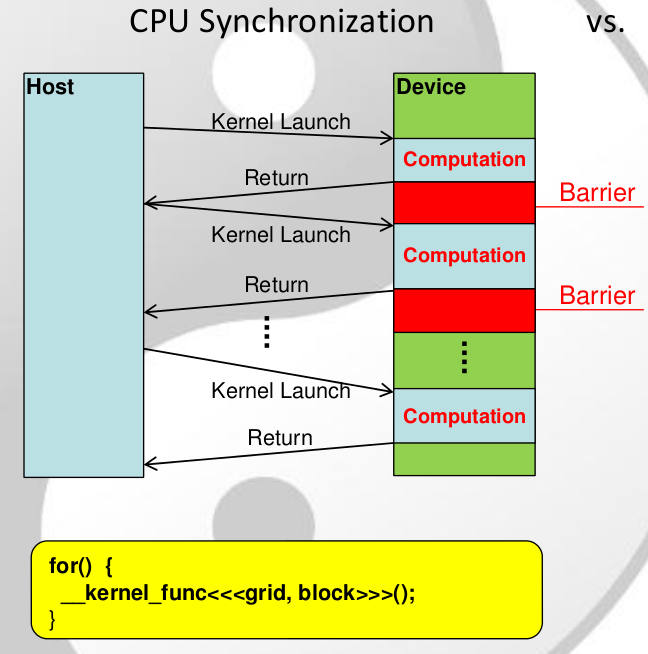
Regarding the second question. Yes, it does declare the amount of shared memory specified per block. Take into account that the quantity of available shared memory is measured per SM. So one should be very careful how the shared memory is used along with the launch configuration.