The exception you get is telling you filedialog
is not in your namespace. filedialog
(and btw messagebox
) is a tkinter module, so it is not imported just with from tkinter import *
>>> from tkinter import * >>> filedialog Traceback (most recent call last): File "<interactive input>", line 1, in <module> NameError: name 'filedialog' is not defined >>>
you should use for example:
>>> from tkinter import filedialog >>> filedialog <module 'tkinter.filedialog' from 'C:\Python32\lib\tkinter\filedialog.py'> >>>
or
>>> import tkinter.filedialog as fdialog
or
>>> from tkinter.filedialog import askopenfilename
So this would do for your browse button:
from tkinter import * from tkinter.filedialog import askopenfilename from tkinter.messagebox import showerror class MyFrame(Frame): def __init__(self): Frame.__init__(self) self.master.title("Example") self.master.rowconfigure(5, weight=1) self.master.columnconfigure(5, weight=1) self.grid(sticky=W+E+N+S) self.button = Button(self, text="Browse", command=self.load_file, width=10) self.button.grid(row=1, column=0, sticky=W) def load_file(self): fname = askopenfilename(filetypes=(("Template files", "*.tplate"), ("HTML files", "*.html;*.htm"), ("All files", "*.*") )) if fname: try: print("""here it comes: self.settings["template"].set(fname)""") except: # <- naked except is a bad idea showerror("Open Source File", "Failed to read file\n'%s'" % fname) return if __name__ == "__main__": MyFrame().mainloop()
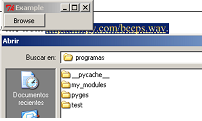