I want to create a monopoly board like
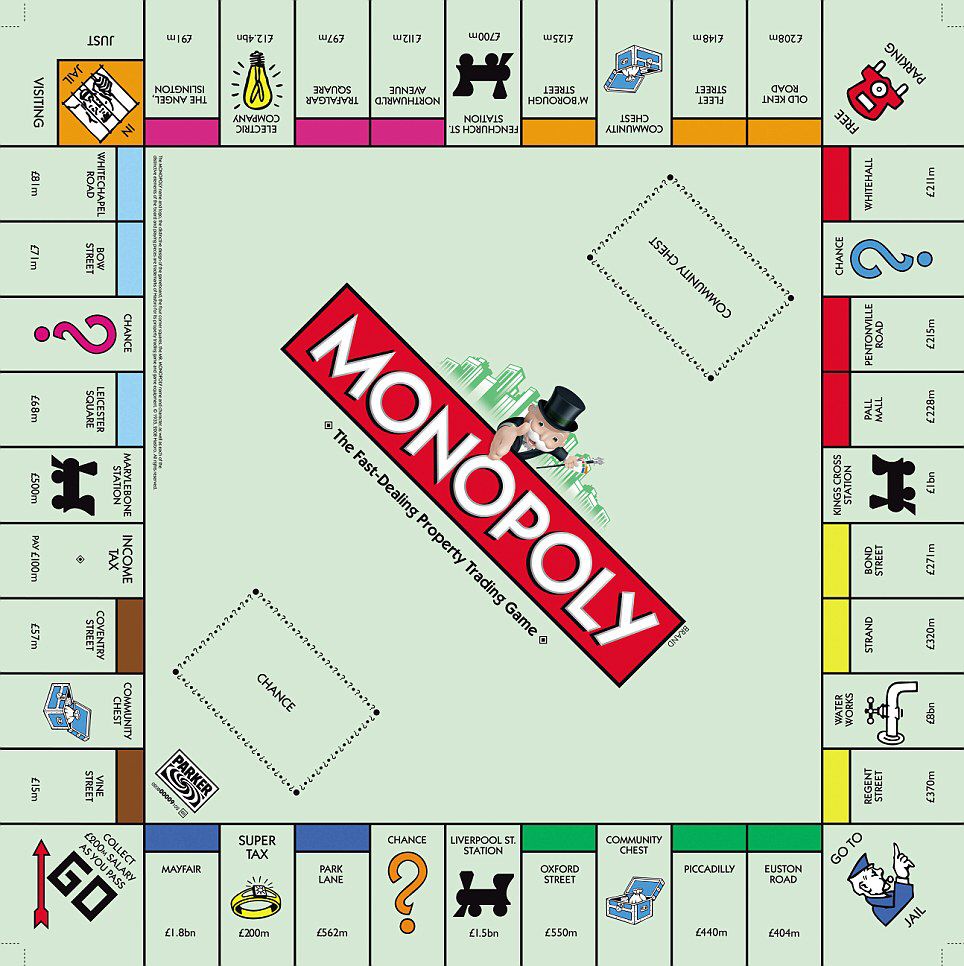
. There are following features in the board
- The corner are square shaped and bigger than other boxes
- The text of each row is facing a specfic angle.
My basic html structure is below
- Board
- Row 1
- Tile 1
- Tile 2 …
- Row 1
I am successful in creating the basic structure using grid-template-areas
. The problem I am facing is that I can’t rotate the tiles of each row according to the need.
I have created a basic snippet which have only 3 tiles per row. The first row is facing right angle all other rows are at wrong angle. 90deg
for second row. 180deg
for third row. and 270deg
for fourth row.
I have tried using writing-mode
and transform:rotate()
but it doesn’t work or maybe I am using it wrong way. Please help me to find the correct way. I will be really thankful
*{ box-sizing: border-box; } #board { display: grid; /*first and last row and column are bigger than others*/ grid-template-columns: 100px repeat(2, 70px) 100px; grid-template-rows: 100px repeat(2, 70px) 100px; /*a, b, c, d are 4 rows and o is center*/ grid-template-areas: "c c c d" "b o o d" "b o o d" "b a a a"; } #center { grid-area: o; } .row { display: flex; } .tile { display: flex; flex-direction: column; border: 1px solid; height: 100%; width: 100%; } .tile-color { flex: 3; background: red; border: 1px solid; } .tile-name { flex: 6; } .tile-price { flex: 3; } /*Flex directions are used to give the tiles correct order*/ #row-0 { grid-area: a; flex-direction: row-reverse; } #row-1 { grid-area: b; flex-direction: column-reverse; } #row-2 { grid-area: c; flex-direction: row; } #row-3 { grid-area: d; flex-direction: column; } /*To make the corner tiles bigger and square*/ .row > .tile:nth-child(1){ flex: 0 0 100px; }
<div id="board"> <div id="center"></div> <!--Row 1--> <div class="row" id="row-0"> <div class="tile"> <div class="tile-name">Go</div> </div> <div class="tile"> <div class="tile-color"></div> <div class="tile-name">Tile 1</div> <div class="tile-price">Price 1</div> </div> <div class="tile"> <div class="tile-color"></div> <div class="tile-name">Tile 2</div> <div class="tile-price">Price 2</div> </div> </div> <!--Row 2--> <div class="row" id="row-1"> <div class="tile"> <div class="tile-name">Just visiting</div> </div> <div class="tile"> <div class="tile-color"></div> <div class="tile-name">Tile 3</div> <div class="tile-price">Price 3</div> </div> <div class="tile"> <div class="tile-color"></div> <div class="tile-name">Tile 4</div> <div class="tile-price">Price 4</div> </div> </div>
<!--Row 3--> <div class="row" id="row-2"> <div class="tile"> <div class="tile-name">Free Parking</div> </div> <div class="tile"> <div class="tile-color"></div> <div class="tile-name">Tile 4</div> <div class="tile-price">Price 4</div> </div> <div class="tile"> <div class="tile-color"></div> <div class="tile-name">Tile 5</div> <div class="tile-price">Price 5</div> </div> </div> <!--Row 4--> <div class="row" id="row-3"> <div class="tile"> <div class="tile-name">Jail</div> </div> <div class="tile"> <div class="tile-color"></div> <div class="tile-name">Tile 6</div> <div class="tile-price">Price 6</div> </div> <div class="tile"> <div class="tile-color"></div> <div class="tile-name">Tile 7</div> <div class="tile-price">Price 7</div> </div> </div> </div>