It means that somewhere in your code, you are calling a function which in turn calls another function and so forth, until you hit the call stack limit.
This is almost always because of a recursive function with a base case that isn’t being met.
Viewing the stack
Consider this code…
(function a() { a(); })();
Here is the stack after a handful of calls…
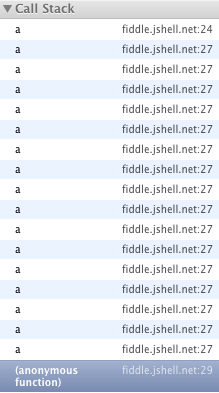
As you can see, the call stack grows until it hits a limit: the browser hardcoded stack size or memory exhaustion.
In order to fix it, ensure that your recursive function has a base case which is able to be met…
(function a(x) { // The following condition // is the base case. if ( ! x) { return; } a(--x); })(10);
Related Posts:
- Video auto play is not working in Safari and Chrome desktop browser
- Failed to load resource: the server responded with a status of 404 (Not Found)
- How to properly use jsPDF library
- Google Chrome Uncaught (in promise) DOMException while playing AUDIO
- ChartJS beginAtZero, min, max doesn’t work
- how to fix : ” TypeError: Cannot read property ‘addEventListener’ of null”…?//
- Cannot set property InnerHTML of null [duplicate]
- ReferenceError: $ is not defined
- React.js: Set innerHTML vs dangerouslySetInnerHTML
- jQuery document.createElement equivalent?
- Pure JavaScript equivalent of jQuery’s $.ready() – how to call a function when the page/DOM is ready for it [duplicate]
- How to play audio?
- How can I scroll to an element using jQuery?
- jQuery.click() vs onClick
- jQuery Get Selected Option From Dropdown
- What is the meaning of == $0 that is shown in inspect element of google chrome for the selected element [duplicate]
- (change) vs (ngModelChange) in angular
- Change Background color (css property) using Jquery
- Failed to load resource: net::ERR_FILE_NOT_FOUND loading json.js
- Get class name using jQuery
- How to clear the canvas for redrawing
- Export html table data to Excel using JavaScript / JQuery is not working properly in chrome browser
- CSS height 100% percent not working
- JavaScript get element by name
- Completely disable scrolling of webpage
- TypeError: Cannot Set property ‘onclick’ of null
- display variable image using cookies
- character counter – backspace doesn’t reflect on characters remaining
- Open URL in same window and in same tab
- Create table with jQuery – append
- How to convert selected HTML to Json?
- How would I call a javascript function in html?
- How to Open New window on every Click
- Encode HTML entities in JavaScript
- “.addEventListener is not a function” why does this error occur?
- Cannot read property ‘getContext’ of null, using canvas
- Coin toss with JavaScript and HTML
- Play an audio file using jQuery when a button is clicked
- How to move an element after another element using JS or jquery?
- How to use Javascript to read local text file and read line by line?
- Why is the jquery script not working?
- getBoundingClientRect is not a function
- Jquery button click() function is not working
- Make iframe automatically adjust height according to the contents without using scrollbar?
- How do I modify the URL without reloading the page?
- Textarea Auto height
- How to add onload event to a div element
- How do I check whether a checkbox is checked in jQuery?
- Print the contents of a DIV
- How to create a HTML Cancel button that redirects to a URL
- What does ngf-select do and why is it needed for form validation?
- Embedding instagram webpage inside an iframe
- How to get the selected radio button’s value?
- why angularjs ng-repeat not working
- Scroll to bottom of div?
- document.getElementById().value doesn’t set the value
- How to run a function when the page is loaded?
- Clearing my form inputs after submission
- Reload an iframe with jQuery
- Highlight a word with jQuery
- How to change the buttons text using javascript
- how to display a div triggered by onclick event
- anchor jumping by using javascript
- jQuery scrollTop() method not working
- FormData append not working
- getContext is not a function
- How to change the text of a label?
- JavaScript – Count Number of Visitor for Website
- Strip HTML from Text JavaScript
- Using “×” word in html changes to ×
- How do I store an array in localStorage?
- Fire oninput event with jQuery
- Changing the selected option of an HTML Select element
- HTML anchor link – href and onclick both?
- How can I know which radio button is selected via jQuery?
- img onclick call to JavaScript function
- How to scroll to top of page with JavaScript/jQuery?
- Calling a JavaScript function in another js file
- How to embed an autoplaying YouTube video in an iframe?
- JavaScript code not running in HTML5 document
- Cannot read property ‘style’ of undefined — Uncaught Type Error
- Unexpected token < in first line of HTML
- How to make HTML element resizable using pure Javascript?
- How to set “style=display:none;” using jQuery’s attr method?
- WordPress Bootstrap Handburger Menu Wont Open
- The value of attribute [ data-type ] must be in double quotes – custom html widget error
- Adding JavaScript to a WordPress website
- Insert “javascript:void(0);” into URL
- How to display post content in the block editor
- My Button Default Click Is Not Working In WordPress Using Javascript Code
- Make a script work within a page
- how to let users upload their custom cover image
- Putting custom html/js page into Elementor as it’s own block
- Add Paypal button redirect to a specific page after completing
- internal anchor links no longer working after upgrade
- Can’t Listen to KeyDown in TInyMCE Iframe (jQuery) and Pass it to Parent HTML FORM
- Making the HTML list to a checkbox tree with the plugin jstree [closed]
- dropdown does not work [closed]
- How do I cycle a JS function in WordPress? [closed]
- I have custom html mixed with inline javascript that I want added to my WordPress site, how do I achieve this?