JavaScript’s implementation of ECMAScript can vary from browser to browser, however for Chrome, many string operations (substr, slice, regex, etc.) simply retain references to the original string rather than making copies of the string. This is a known issue in Chrome (Bug #2869). To force a copy of the string, the following code works:
var string_copy = (' ' + original_string).slice(1);
This code works by appending a space to the front of the string. This concatenation results in a string copy in Chrome’s implementation. Then the substring after the space can be referenced.
This problem with the solution has been recreated here: http://jsfiddle.net/ouvv4kbs/1/
WARNING: takes a long time to load, open Chrome debug console to see a progress printout.
// We would expect this program to use ~1 MB of memory, however taking // a Heap Snapshot will show that this program uses ~100 MB of memory. // If the processed data size is increased to ~1 GB, the Chrome tab // will crash due to running out of memory. function randomString(length) { var alphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'; var result = ''; for (var i = 0; i < length; i++) { result += alphabet[Math.round(Math.random() * (alphabet.length - 1))]; } return result; }; var substrings = []; var extractSubstring = function(huge_string) { var substring = huge_string.substr(0, 100 * 1000 /* 100 KB */); // Uncommenting this line will force a copy of the string and allow // the unused memory to be garbage collected // substring = (' ' + substring).slice(1); substrings.push(substring); }; // Process 100 MB of data, but only keep 1 MB. for (var i = 0; i < 10; i++) { console.log(10 * (i + 1) + 'MB processed'); var huge_string = randomString(10 * 1000 * 1000 /* 10 MB */); extractSubstring(huge_string); } // Do something which will keep a reference to substrings around and // prevent it from being garbage collected. setInterval(function() { var i = Math.round(Math.random() * (substrings.length - 1)); document.body.innerHTML = substrings[i].substr(0, 10); }, 2000);
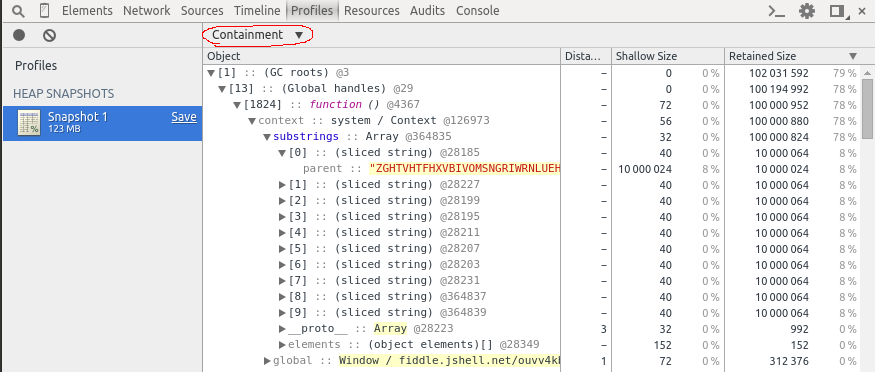