You can use canvas’ context.translate & context.rotate to do rotate your image
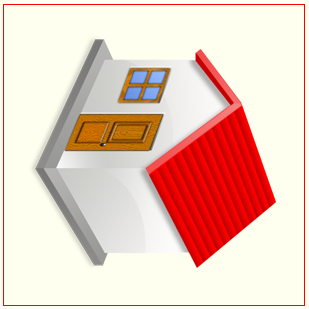
Here’s a function to draw an image that is rotated by the specified degrees:
function drawRotated(degrees){ context.clearRect(0,0,canvas.width,canvas.height); // save the unrotated context of the canvas so we can restore it later // the alternative is to untranslate & unrotate after drawing context.save(); // move to the center of the canvas context.translate(canvas.width/2,canvas.height/2); // rotate the canvas to the specified degrees context.rotate(degrees*Math.PI/180); // draw the image // since the context is rotated, the image will be rotated also context.drawImage(image,-image.width/2,-image.width/2); // we’re done with the rotating so restore the unrotated context context.restore(); }
Here is code and a Fiddle: http://jsfiddle.net/m1erickson/6ZsCz/
<!doctype html> <html> <head> <link rel="stylesheet" type="text/css" media="all" href="css/reset.css" /> <!-- reset css --> <script type="text/javascript" src="http://code.jquery.com/jquery.min.js"></script> <style> body{ background-color: ivory; } canvas{border:1px solid red;} </style> <script> $(function(){ var canvas=document.getElementById("canvas"); var ctx=canvas.getContext("2d"); var angleInDegrees=0; var image=document.createElement("img"); image.onload=function(){ ctx.drawImage(image,canvas.width/2-image.width/2,canvas.height/2-image.width/2); } image.src="houseicon.png"; $("#clockwise").click(function(){ angleInDegrees+=30; drawRotated(angleInDegrees); }); $("#counterclockwise").click(function(){ angleInDegrees-=30; drawRotated(angleInDegrees); });