There is a (somewhat) related question on StackOverflow:
Here the problem was that an array of shape (nx,ny,1) is still considered a 3D array, and must be squeeze
d or sliced into a 2D array.
More generally, the reason for the Exception
TypeError: Invalid dimensions for image data
is shown here: matplotlib.pyplot.imshow()
needs a 2D array, or a 3D array with the third dimension being of shape 3 or 4!
You can easily check this with (these checks are done by imshow
, this function is only meant to give a more specific message in case it’s not a valid input):
from __future__ import print_function import numpy as np def valid_imshow_data(data): data = np.asarray(data) if data.ndim == 2: return True elif data.ndim == 3: if 3 <= data.shape[2] <= 4: return True else: print('The "data" has 3 dimensions but the last dimension ' 'must have a length of 3 (RGB) or 4 (RGBA), not "{}".' ''.format(data.shape[2])) return False else: print('To visualize an image the data must be 2 dimensional or ' '3 dimensional, not "{}".' ''.format(data.ndim)) return False
In your case:
>>> new_SN_map = np.array([1,2,3]) >>> valid_imshow_data(new_SN_map) To visualize an image the data must be 2 dimensional or 3 dimensional, not "1". False
The np.asarray
is what is done internally by matplotlib.pyplot.imshow
so it’s generally best you do it too. If you have a numpy array it’s obsolete but if not (for example a list
) it’s necessary.
In your specific case you got a 1D array, so you need to add a dimension with np.expand_dims()
import matplotlib.pyplot as plt a = np.array([1,2,3,4,5]) a = np.expand_dims(a, axis=0) # or axis=1 plt.imshow(a) plt.show()
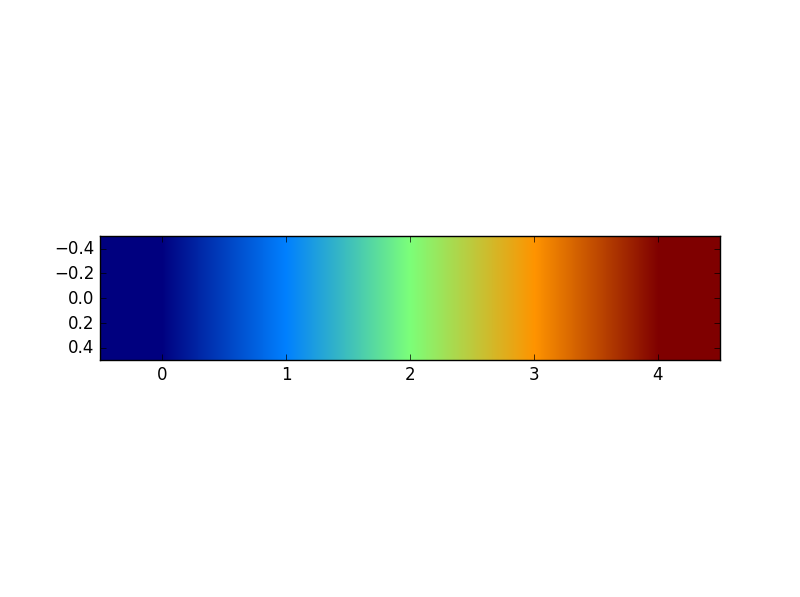
or just use something that accepts 1D arrays like plot
:
a = np.array([1,2,3,4,5]) plt.plot(a) plt.show()
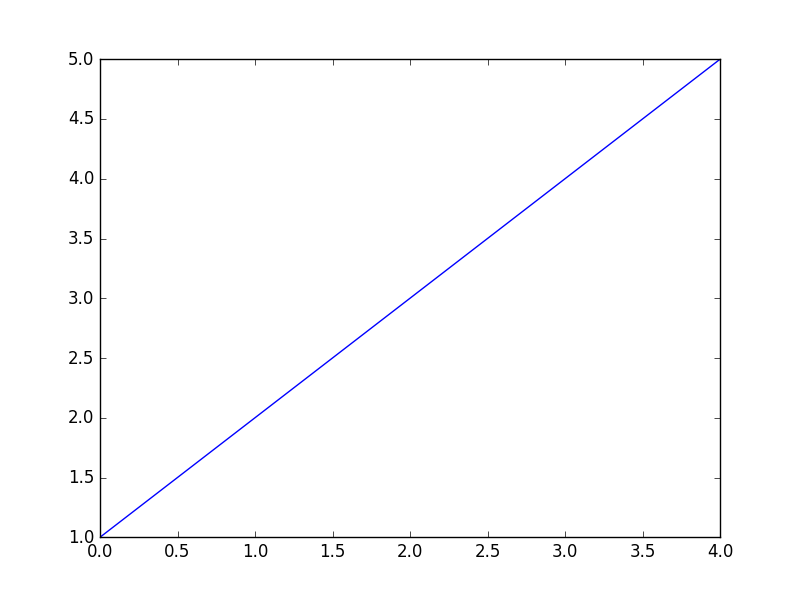