arange
generates lists (well, numpy arrays); type help(np.arange)
for the details. You don’t need to call it on existing lists.
>>> x = [1,2,3,4] >>> y = [3,5,7,9] >>> >>> m,b = np.polyfit(x, y, 1) >>> m 2.0000000000000009 >>> b 0.99999999999999833
I should add that I tend to use poly1d
here rather than write out “m*x+b” and the higher-order equivalents, so my version of your code would look something like this:
import numpy as np import matplotlib.pyplot as plt x = [1,2,3,4] y = [3,5,7,10] # 10, not 9, so the fit isn't perfect coef = np.polyfit(x,y,1) poly1d_fn = np.poly1d(coef) # poly1d_fn is now a function which takes in x and returns an estimate for y plt.plot(x,y, 'yo', x, poly1d_fn(x), '--k') #'--k'=black dashed line, 'yo' = yellow circle marker plt.xlim(0, 5) plt.ylim(0, 12)
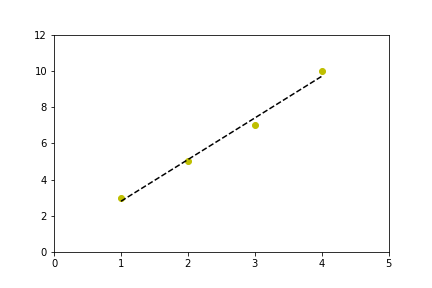
Related Posts:
- ImportError: DLL load failed: The specified module could not be found
- ImportError: DLL load failed: The specified module could not be found
- Plotting a 2D heatmap with Matplotlib
- Unable to plot Double Bar, Bar plot using pyplot for ndarray
- TypeError: ‘DataFrame’ object is not callable
- Overcome ValueError for empty array
- ValueError: x and y must be the same size
- TypeError: Invalid dimensions for image data when plotting array with imshow()
- Matplotlib: TypeError: can’t multiply sequence by non-int of type ‘numpy.float64’
- How to plot an array in python?
- IndexError: index 2 is out of bounds for axis 0 with size 2
- TypeError: zip argument #2 must support iteration
- numpy/scipy/ipython:Failed to interpret file as a pickle
- ValueError: setting an array element with a sequence
- TypeError: only size-1 arrays can be converted to Python scalars (matplotlib)
- What exactly does numpy.exp() do? [closed]
- How do you change the size of figures drawn with Matplotlib?
- numpy: Invalid value encountered in true_divide
- Is “from matplotlib import pyplot as plt” == “import matplotlib.pyplot as plt”?
- python numpy ValueError: operands could not be broadcast together with shapes
- _tkinter.TclError: no display name and no $DISPLAY environment variable
- How to fix IndexError: invalid index to scalar variable
- Could not install packages due to a “Environment error :[error 13]: permission denied : ‘usr/local/bin/f2py'”
- How does numpy.newaxis work and when to use it?
- Should I use np.absolute or np.abs?
- How to plot a histogram using Matplotlib in Python with a list of data?
- Convert pandas dataframe to NumPy array
- In Matplotlib, what does the argument mean in fig.add_subplot(111)?
- Error: all the input array dimensions except for the concatenation axis must match exactly
- matplotlib error – no module named tkinter
- Singular matrix issue with Numpy
- How to find all occurrences of an element in a list
- TypeError: ‘numpy.float64’ object is not callable
- ‘DataFrame’ object has no attribute ‘sort’
- ValueError: all the input arrays must have same number of dimensions
- TypeError: cannot unpack non-iterable int objec
- ValueError: setting an array element with a sequence
- How do I set the figure title and axes labels font size in Matplotlib?
- How to raise a numpy array to a power? (corresponding to repeated matrix multiplications, not elementwise)
- Is there a list of line styles in matplotlib?
- ‘End of statement expected’ in pycharm
- What does numpy.gradient do?
- Plotting multiple different plots in one figure using Seaborn
- Change figure size and figure format in matplotlib
- ‘list’ object has no attribute ‘shape’
- load csv into 2D matrix with numpy for plotting
- Purpose of `numpy.log1p( )`?
- How to take column-slices of dataframe in pandas
- Using %matplotlib notebook after %matplotlib inline in Jupyter Notebook doesn’t work
- index 1 is out of bounds for axis 0 with size 1
- Display image as grayscale using matplotlib
- Pytorch reshape tensor dimension
- Most efficient way to reverse a numpy array
- How to remove specific elements in a numpy array
- Overflow / math range error for log or exp
- Creating a Pandas DataFrame from a Numpy array: How do I specify the index column and column headers?
- Overflow Error in Python’s numpy.exp function
- Modify the legend of pandas bar plot
- Plot a horizontal line using matplotlib
- How to normalize a 2-dimensional numpy array in python less verbose?
- Remove xticks in a matplotlib plot?
- How to add title to seaborn boxplot
- Understanding NumPy’s einsum
- For loop and ‘numpy.float64’ object is not iterable error
- What are the causes of overflow encountered in double_scalars besides division by zero?
- RuntimeWarning: divide by zero encountered in log
- How to update a plot in matplotlib?
- RuntimeWarning: numpy.dtype size changed, may indicate binary incompatibility
- Error NameError: name ‘np’ is not defined
- How to change plot background color?
- raise LinAlgError(“SVD did not converge”) LinAlgError: SVD did not converge in matplotlib pca determination
- Numpy.dot TypeError: Cannot cast array data from dtype(‘float64’) to dtype(‘S32’) according to the rule ‘safe’
- NumPy array is not JSON serializable
- Moving average or running mean
- How to start from second index for for-loop
- Using numpy to build an array of all combinations of two arrays
- Can’t update to numpy 1.13 with anaconda?
- TypeError: only integer arrays with one element can be converted to an index 3
- How to create a numpy array of all True or all False?
- Removing nan values from an array
- LinAlgError: Last 2 dimensions of the array must be square
- Building multi-regression model throws error: `Pandas data cast to numpy dtype of object. Check input data with np.asarray(data).`
- Nonlinear regression with python – what’s a simple method to fit this data better?
- ImportError in importing from sklearn: cannot import name check_build
- Root mean square of a function in python
- What are the differences between numpy arrays and matrices? Which one should I use?
- Plot a histogram such that the total area of the histogram equals 1
- How to change legend size with matplotlib.pyplot
- An equivalent function to matplotlib.mlab.bivariate_normal
- mean, nanmean and warning: Mean of empty slice
- Is there any numpy group by function?
- Replacing Pandas or Numpy Nan with a None to use with MysqlDB
- Plotting a python dict in order of key values
- How to normalize a NumPy array to within a certain range?
- How to implement the ReLU function in Numpy
- MovieWriter ffmpeg unavailable; trying to use class ‘matplotlib.animation.PillowWriter’ instead
- Sorting arrays in NumPy by column
- numpy : calculate the derivative of the softmax function
- Conditional indexing with Numpy ndarray
- python numpy machine epsilon