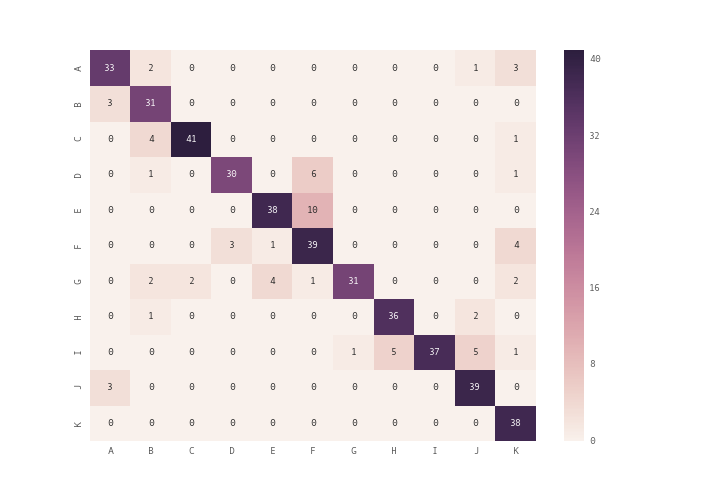
you can use plt.matshow()
instead of plt.imshow()
or you can use seaborn module’s heatmap
(see documentation) to plot the confusion matrix
import seaborn as sn import pandas as pd import matplotlib.pyplot as plt array = [[33,2,0,0,0,0,0,0,0,1,3], [3,31,0,0,0,0,0,0,0,0,0], [0,4,41,0,0,0,0,0,0,0,1], [0,1,0,30,0,6,0,0,0,0,1], [0,0,0,0,38,10,0,0,0,0,0], [0,0,0,3,1,39,0,0,0,0,4], [0,2,2,0,4,1,31,0,0,0,2], [0,1,0,0,0,0,0,36,0,2,0], [0,0,0,0,0,0,1,5,37,5,1], [3,0,0,0,0,0,0,0,0,39,0], [0,0,0,0,0,0,0,0,0,0,38]] df_cm = pd.DataFrame(array, index = [i for i in "ABCDEFGHIJK"], columns = [i for i in "ABCDEFGHIJK"]) plt.figure(figsize = (10,7)) sn.heatmap(df_cm, annot=True)
Related Posts:
- ImportError: DLL load failed: The specified module could not be found
- ImportError: DLL load failed: The specified module could not be found
- TypeError: only size-1 arrays can be converted to Python scalars (matplotlib)
- Showing an image with pylab.imshow()
- How do you change the size of figures drawn with Matplotlib?
- No module named ‘sklearn.cross_validation’
- Is “from matplotlib import pyplot as plt” == “import matplotlib.pyplot as plt”?
- _tkinter.TclError: no display name and no $DISPLAY environment variable
- ValueError: operands could not be broadcast together with shapes (5,) (30,)
- ValueError: Unknown label type: ‘continuous’
- _tkinter.TclError: no display name and no $DISPLAY environment variable
- ImportError: No module named matplotlib.pyplot
- Transpose/Unzip Function (inverse of zip)?
- numpy matrix vector multiplication
- sklearn error ValueError: Input contains NaN, infinity or a value too large for dtype(‘float64’)
- sklearn error ValueError: Input contains NaN, infinity or a value too large for dtype(‘float64’)
- TypeError: cannot perform reduce with flexible type
- data type not understood
- Plotting a 2D heatmap with Matplotlib
- Save plot to image file instead of displaying it using Matplotlib
- ModuleNotFoundError: No module named ‘sklearn’
- How to plot a histogram using Matplotlib in Python with a list of data?
- In Matplotlib, what does the argument mean in fig.add_subplot(111)?
- ModuleNotFoundError: No module named ‘sklearn’
- pandas DataFrame “no numeric data to plot” error
- How to change the font size on a matplotlib plot
- Permission denied error by installing matplotlib
- Updating matplotlib in virtualenv with pip
- How to normalize a NumPy array to a unit vector?
- How do I set the figure title and axes labels font size in Matplotlib?
- How to customize a scatter matrix to see all titles?
- Matplotlib automatic legend outside plot
- How to get element-wise matrix multiplication (Hadamard product) in numpy?
- Matplotlib automatic legend outside plot
- Changing the “tick frequency” on x or y axis in matplotlib?
- matplotlib error – no module named tkinter
- Plot pie chart and table of pandas dataframe
- ModuleNotFoundError: No module named ‘matplotlib’
- Add Legend to Seaborn point plot
- TypeError: ‘DataFrame’ object is not callable
- How do I plot only a table in Matplotlib?
- Python equivalent to ‘hold on’ in Matlab
- Inverse of a matrix using numpy
- How to add title to subplots in Matplotlib
- How to change a ‘LinearSegmentedColormap’ to a different distribution of color?
- Create own colormap using matplotlib and plot color scale
- How to increase plt.title font size?
- How to make a 3D scatter plot in matplotlib
- Arrays used as indices must be of integer (or boolean) type
- How to draw vertical lines on a given plot in matplotlib
- Modify the legend of pandas bar plot
- Changing the “tick frequency” on x or y axis in matplotlib
- How can I set the aspect ratio in matplotlib?
- Remove xticks in a matplotlib plot?
- python SyntaxError: invalid syntax %matplotlib inline
- sklearn Logistic Regression “ValueError: Found array with dim 3. Estimator expected <= 2."
- RuntimeWarning: numpy.dtype size changed, may indicate binary incompatibility
- How to make a histogram from a list of data
- How do I change the figure size with subplots?
- ValueError: x and y must be the same size
- raise LinAlgError(“SVD did not converge”) LinAlgError: SVD did not converge in matplotlib pca determination
- TypeError: Invalid dimensions for image data when plotting array with imshow()
- matplotlib does not show my drawings although I call pyplot.show()
- Linear regression with matplotlib / numpy
- Get Confusion Matrix From a Keras Multiclass Model
- What’s the difference between scikit-learn and tensorflow? Is it possible to use them together?
- How to save a Seaborn plot into a file
- Scatter plot colorbar – Matplotlib
- matplotlib: how to draw a rectangle on image
- bbox_to_anchor and loc in matplotlib
- How to put the legend out of the plot
- Matplotlib: TypeError: can’t multiply sequence by non-int of type ‘numpy.float64’
- RuntimeError: Invalid DISPLAY variable
- How to plot an array in python?
- Scikit-learn GridSearch giving “ValueError: multiclass format is not supported” error
- When I use matplotlib in jupyter notebook,it always raise ” matplotlib is currently using a non-GUI backend” error?
- Python 3: Multiply a vector by a matrix without NumPy
- IndexError: index 2 is out of bounds for axis 0 with size 2
- ImportError in importing from sklearn: cannot import name check_build
- Can sklearn random forest directly handle categorical features?
- What are the differences between numpy arrays and matrices? Which one should I use?
- Why do many examples use `fig, ax = plt.subplots()` in Matplotlib/pyplot/python
- ImportError: No module named model_selection
- Plot a histogram such that the total area of the histogram equals 1
- How to change legend size with matplotlib.pyplot
- An equivalent function to matplotlib.mlab.bivariate_normal
- plot a circle with pyplot
- how to check which version of nltk, scikit learn installed?
- IPython, “name ‘plt’ not defined”
- Plotting a python dict in order of key values
- Convert a 1D array to a 2D array in numpy
- Pandas dataframe groupby plot
- MovieWriter ffmpeg unavailable; trying to use class ‘matplotlib.animation.PillowWriter’ instead
- Python/Scikit-Learn – Can’t handle mix of multiclass and continuous
- How do I tell Matplotlib to create a second (new) plot, then later plot on the old one?
- ValueError: multiclass format is not supported
- Conditional indexing with Numpy ndarray
- Equivalent to matlab’s imagesc in matplotlib? [duplicate]
- numpy/scipy/ipython:Failed to interpret file as a pickle
- alueError: ordinal must be >= 1