If you just wanted to change the example and put the table at the top, then loc='top'
in the table declaration is what you need,
the_table = ax.table(cellText=cell_text, rowLabels=rows, rowColours=colors, colLabels=columns, loc='top')
Then adjusting the plot with,
plt.subplots_adjust(left=0.2, top=0.8)
A more flexible option is to put the table in its own axis using subplots,
import numpy as np import matplotlib.pyplot as plt fig, axs =plt.subplots(2,1) clust_data = np.random.random((10,3)) collabel=("col 1", "col 2", "col 3") axs[0].axis('tight') axs[0].axis('off') the_table = axs[0].table(cellText=clust_data,colLabels=collabel,loc='center') axs[1].plot(clust_data[:,0],clust_data[:,1]) plt.show()
which looks like this,
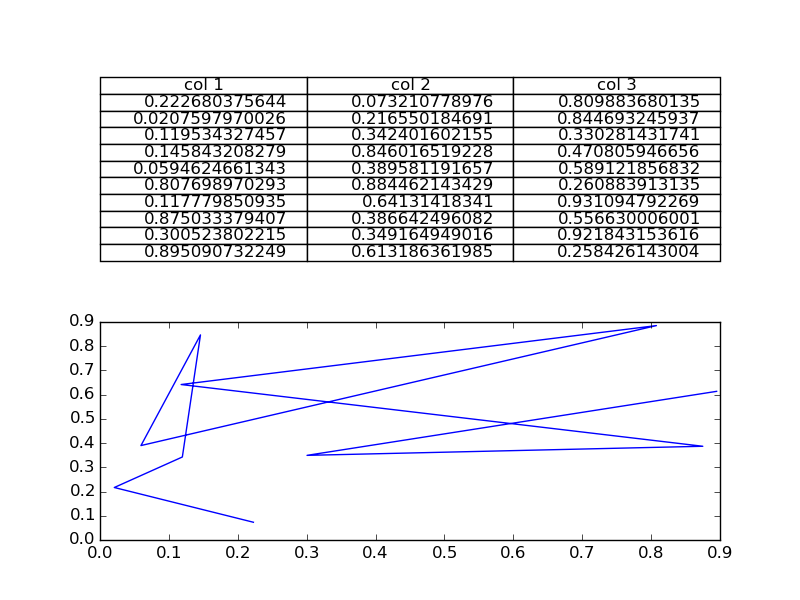
You are then free to adjust the locations of the axis as required.